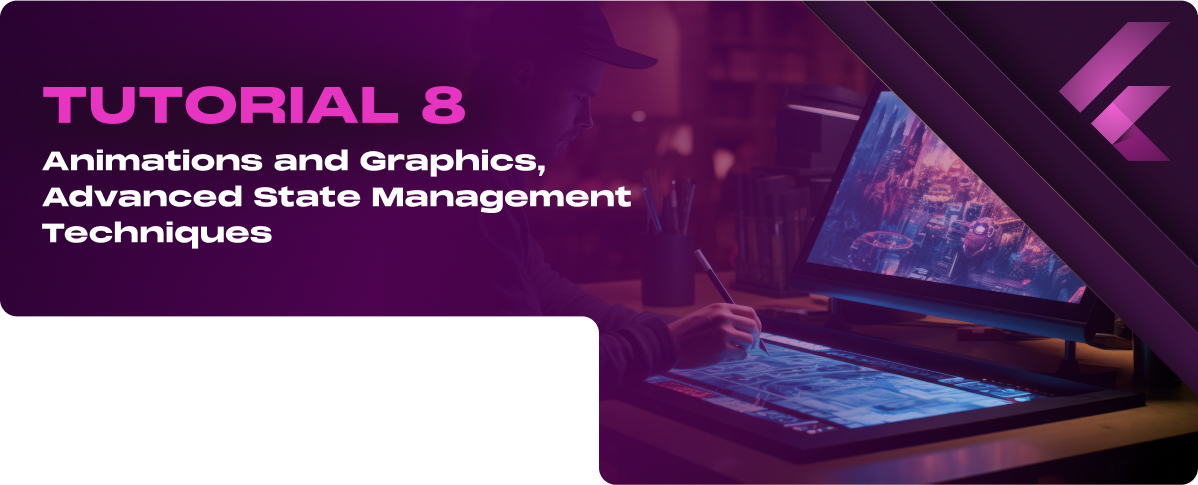
Tutorial 8: Animations and Graphics, Advanced State Management Techniques
RELATED TUTORIALS
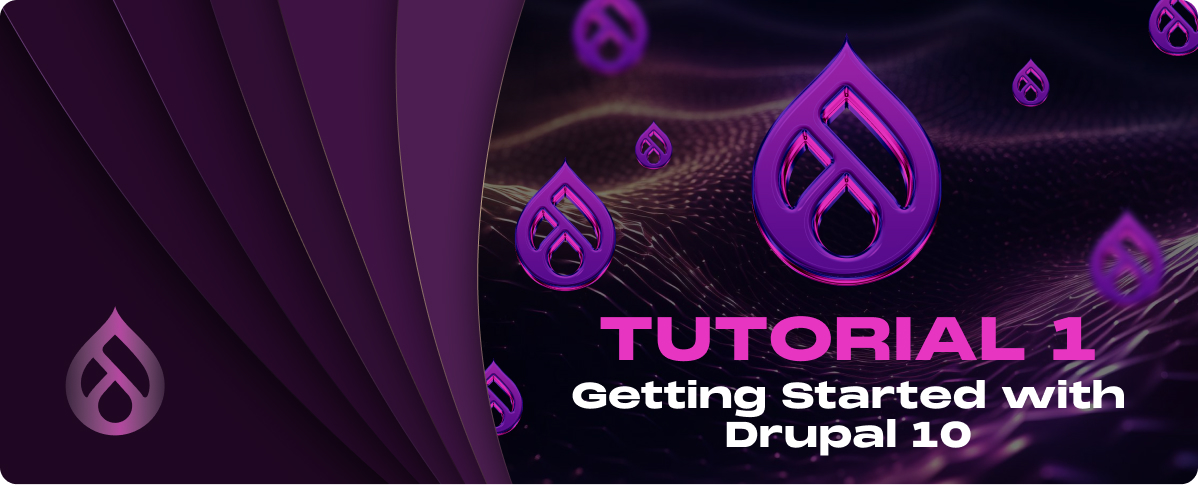
Tutorial 1: Getting Started with Drupal 10
Introduction Drupal is an open-source content management system that powers millions of websites and applications. It's built, used,…
Read More
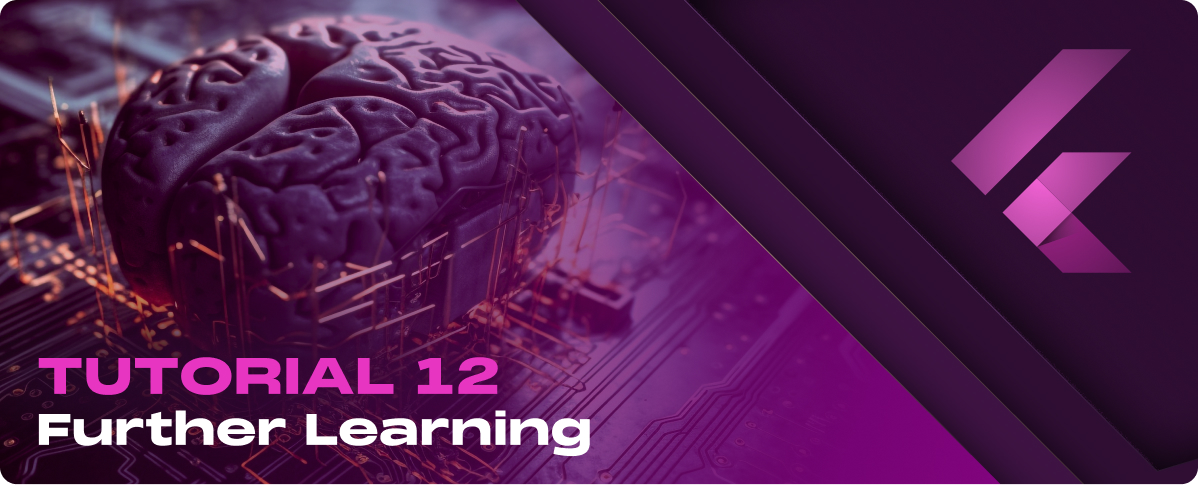
Tutorial 12: Further Learning
Resources for Further Learning Official Documentation The Flutter team provides comprehensive documentation covering every aspect of Flutter development,…
Read More
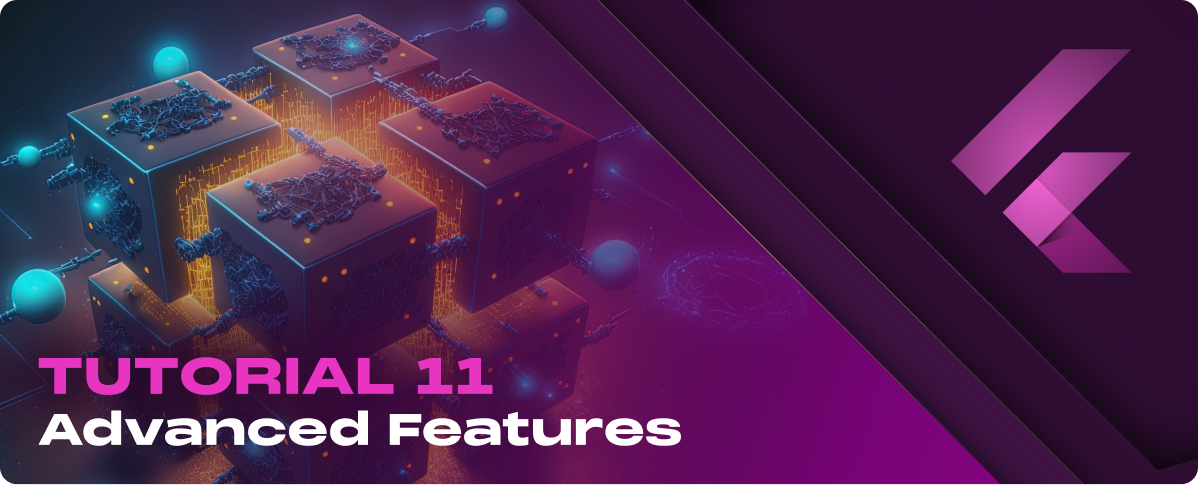
Tutorial 11: Advanced Features
Advanced UI Components and Techniques Custom Widgets and Reusability Creating custom widgets helps encapsulate and abstract complex UI…
Read More
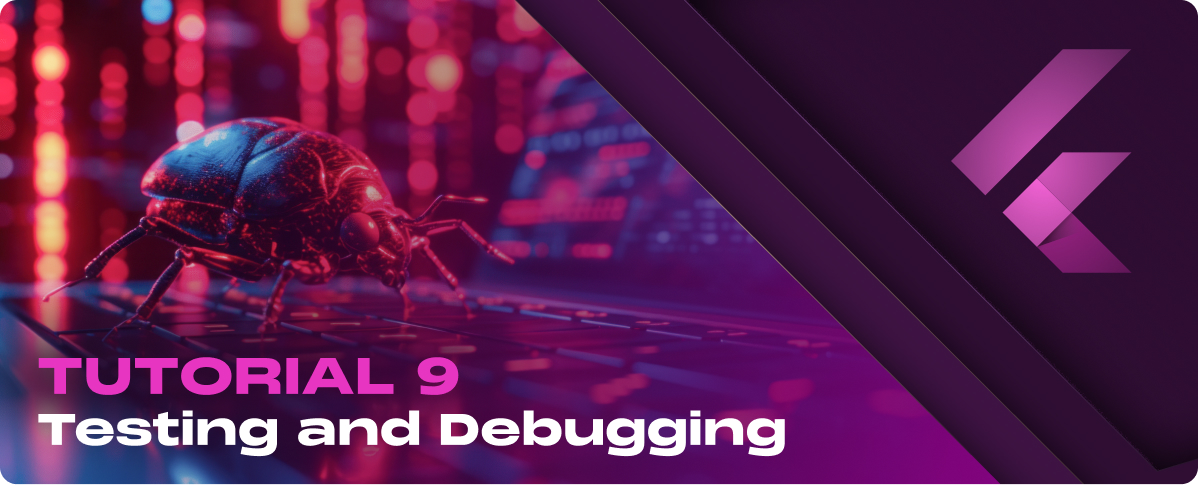
Tutorial 9: Testing and Debugging
Writing Unit and Widget Tests Introduction to Testing in Flutter Flutter provides a comprehensive framework for automated testing,…
Read More
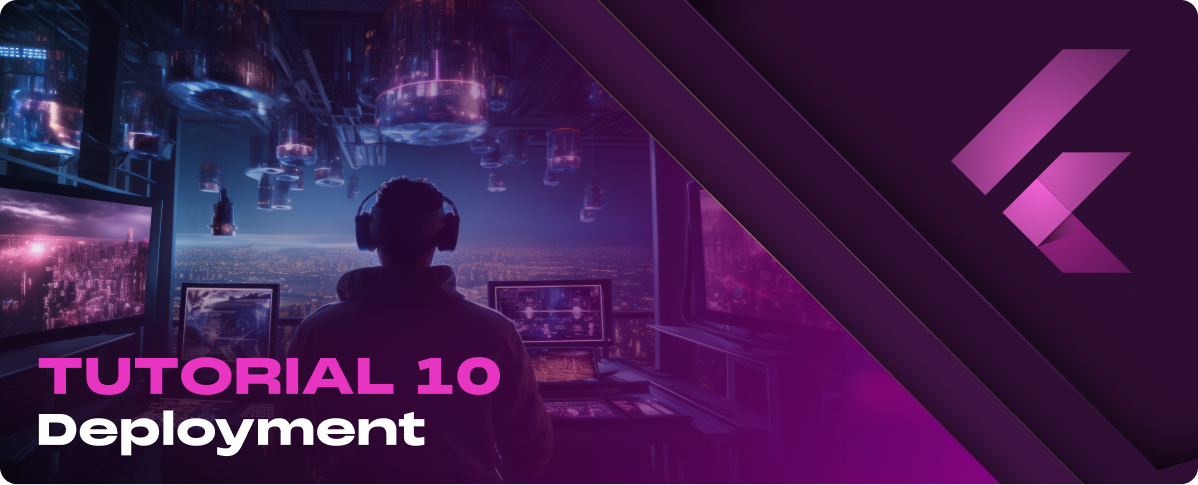
Tutorial 10: Deployment
Preparing for Release Optimizing Performance Before releasing your app, optimizing its performance is important to ensure a smooth…