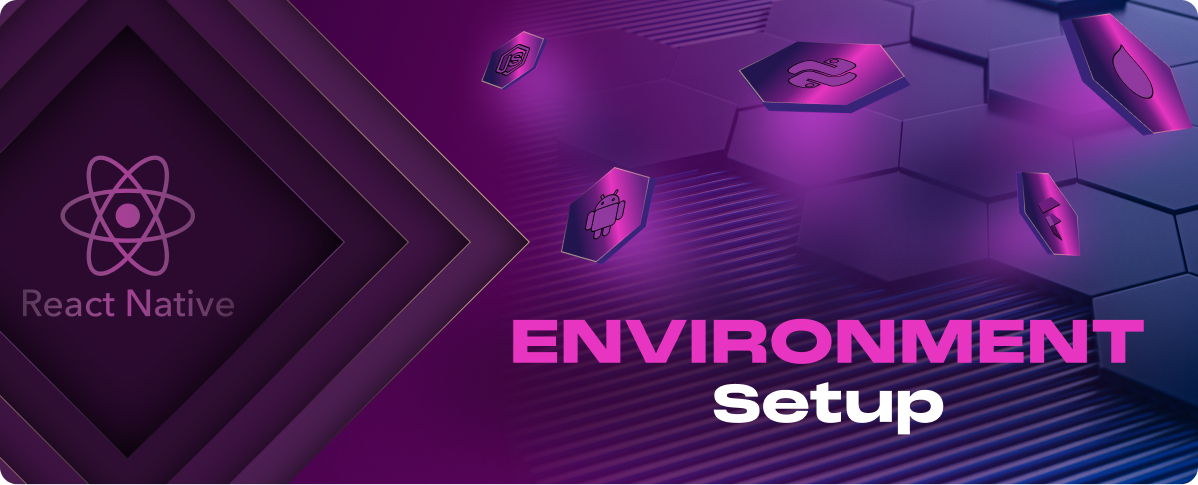
Tutorial 1: React Native – Environment Setup
RELATED TUTORIALS
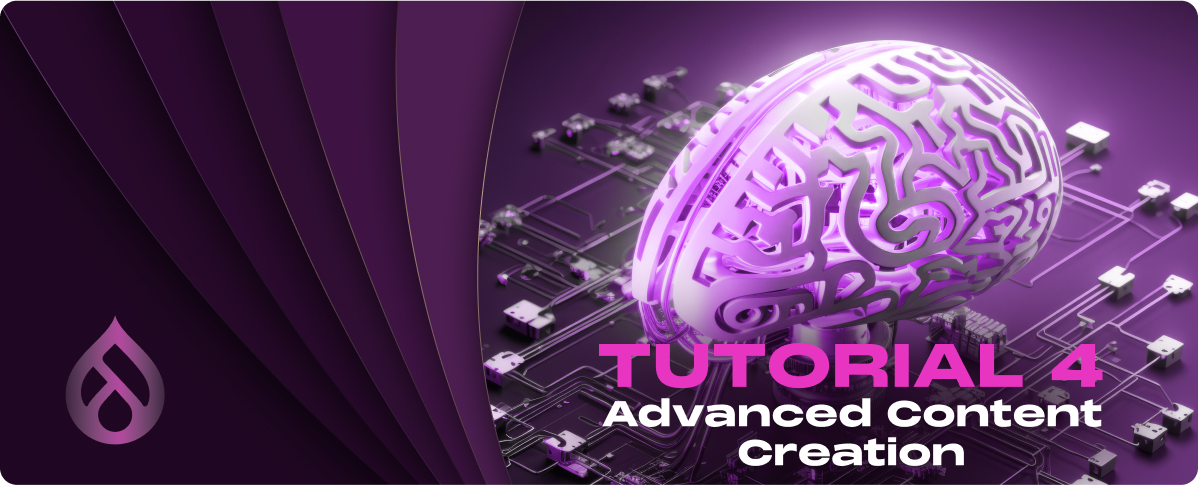
Tutorial 4: Advanced Content Creation in Drupal
Creating Articles in Drupal Articles in Drupal are a content type with a predefined set of fields that…
Read More
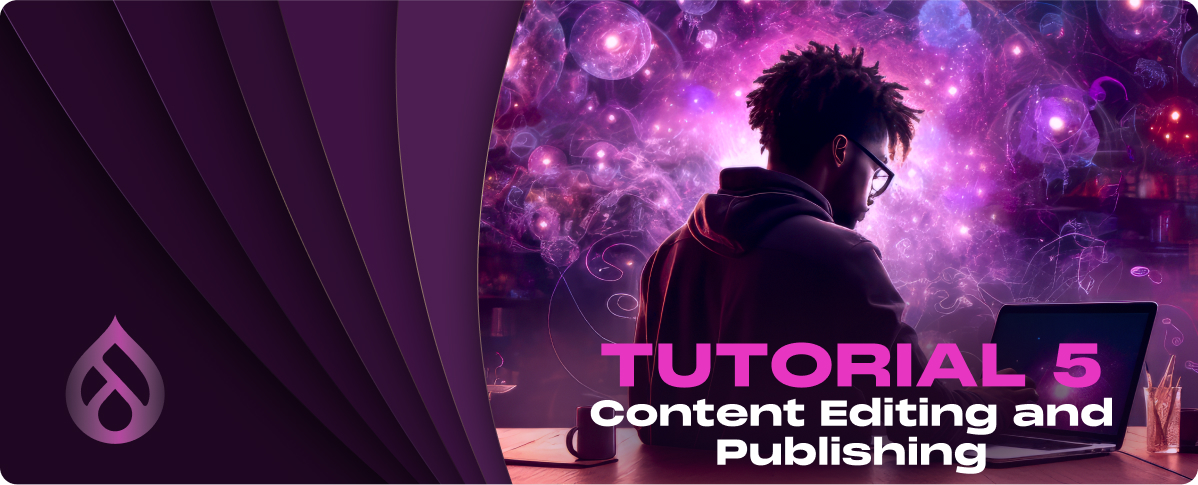
Tutorial 5: Content Editing and Publishing in Drupal
Modifying Content in Drupal Keeping your website's content updated is important for maintaining its relevance and accuracy. Drupal's…
Read More
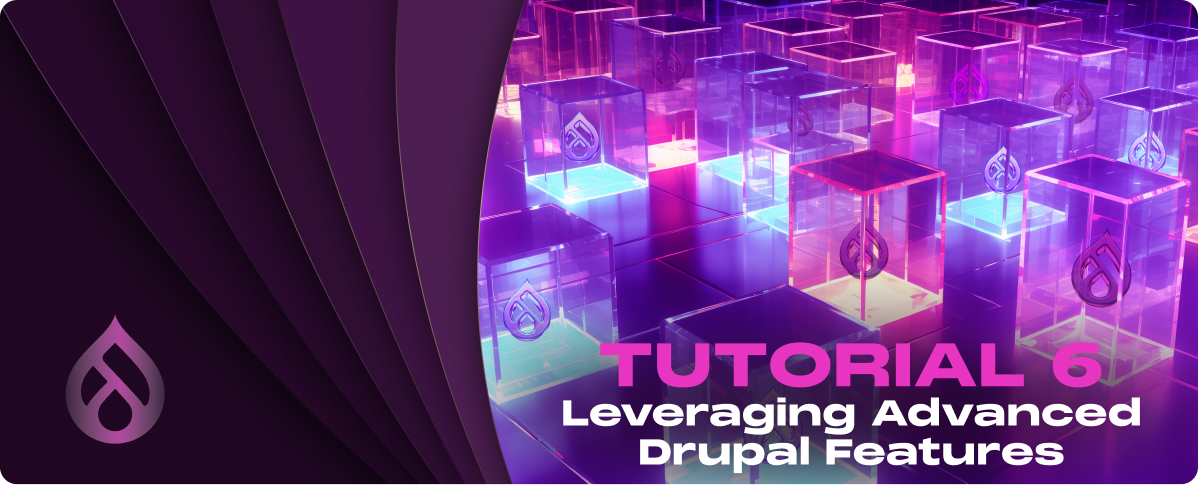
Tutorial 6: Leveraging Advanced Drupal Features in Drupal
Web Services in Drupal Drupal 8 and later versions come with significant support for web services, making it…
Read More
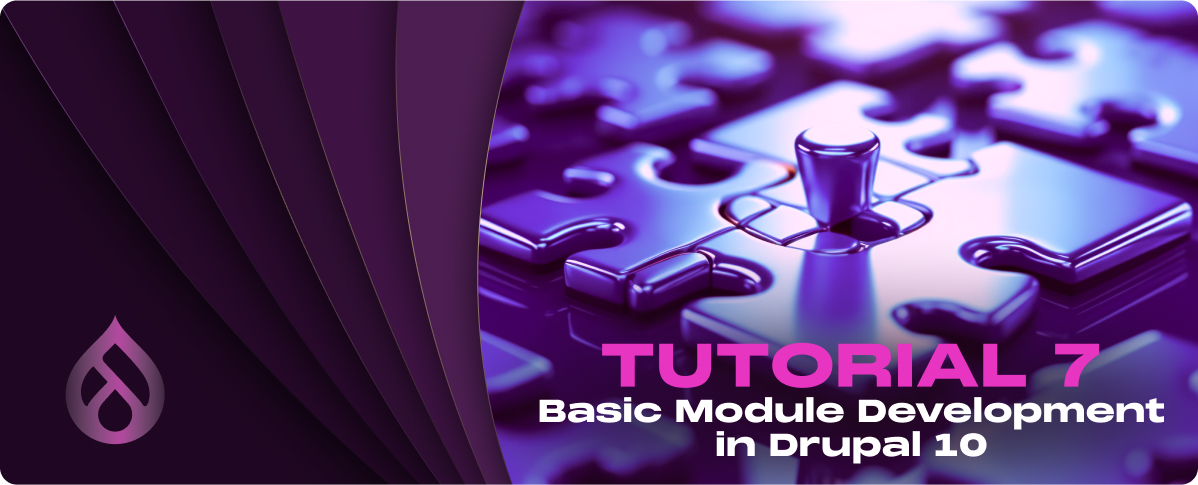
Tutorial 7: Basic Module Development in Drupal 10
Introduction to Modules in Drupal Modules are packages of PHP code that extend Drupal's core capabilities. They can…
Read More
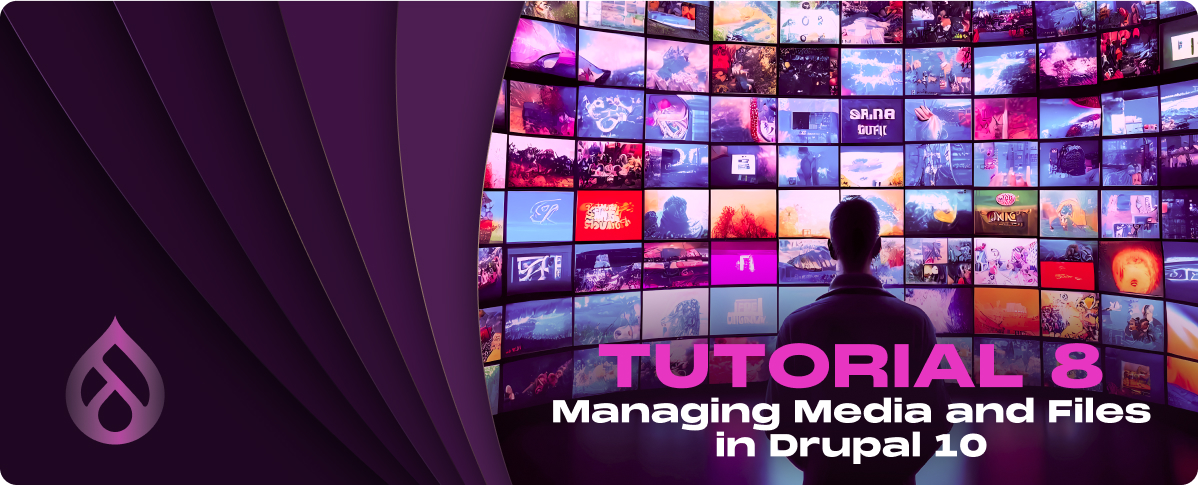
Tutorial 8: Managing Media and Files in Drupal…
Uploading and Managing Media Drupal's Media Library offers an integrated solution to handle media assets, allowing you to…